Forecasting with Exogenous Regressors¶
This notebook provides examples of the accepted data structures for passing the expected value of exogenous variables when these are included in the mean. For example, consider an AR(1) with 2 exogenous variables. The mean dynamics are
The \(h\)-step forecast, \(E_{T}[Y_{t+h}]\), depends on the conditional expectation of \(X_{0,T+h}\) and \(X_{1,T+h}\),
where \(E_{T}[Y_{T+h-1}]\) has been recursively computed.
In order to construct forecasts up to some horizon \(h\), it is necessary to pass \(2\times h\) values (\(h\) for each series). If using the features of forecast
that allow many forecast to be specified, it necessary to supply \(n \times 2 \times h\) values.
There are two general purpose data structures that can be used for any number of exogenous variables and any number steps ahead:
dict
- The values can be pass using adict
where the keys are the variable names and the values are 2-dimensional arrays. This is the most natural generalization of a pandasDataFrame
to 3-dimensions.array
- The vales can alternatively be passed as a 3-d NumPyarray
where dimension 0 tracks the regressor index, dimension 1 is the time period and dimension 2 is the horizon.
When a model contains a single exogenous regressor it is possible to use a 2-d array or DataFrame
where dim0 tracks the time period where the forecast is generated and dimension 1 tracks the horizon.
In the special case where a model contains a single regressor and the horizon is 1, then a 1-d array or pandas Series can be used.
[1]:
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import seaborn
seaborn.set_style("darkgrid")
plt.rc("figure", figsize=(16, 6))
plt.rc("savefig", dpi=90)
plt.rc("font", family="sans-serif")
plt.rc("font", size=14)
Simulating data¶
Two \(X\) variables are simulated and are assumed to follow independent AR(1) processes. The data is then assumed to follow an ARX(1) with 2 exogenous regressors and GARCH(1,1) errors.
[2]:
from arch.univariate import ARX, GARCH, ZeroMean, arch_model
burn = 250
x_mod = ARX(None, lags=1)
x0 = x_mod.simulate([1, 0.8, 1], nobs=1000 + burn).data
x1 = x_mod.simulate([2.5, 0.5, 1], nobs=1000 + burn).data
resid_mod = ZeroMean(volatility=GARCH())
resids = resid_mod.simulate([0.1, 0.1, 0.8], nobs=1000 + burn).data
phi1 = 0.7
phi0 = 3
y = 10 + resids.copy()
for i in range(1, y.shape[0]):
y[i] = phi0 + phi1 * y[i - 1] + 2 * x0[i] - 2 * x1[i] + resids[i]
x0 = x0.iloc[-1000:]
x1 = x1.iloc[-1000:]
y = y.iloc[-1000:]
y.index = x0.index = x1.index = np.arange(1000)
Plotting the data¶
[3]:
ax = pd.DataFrame({"ARX": y}).plot(legend=False)
ax.legend(frameon=False)
_ = ax.set_xlim(0, 999)
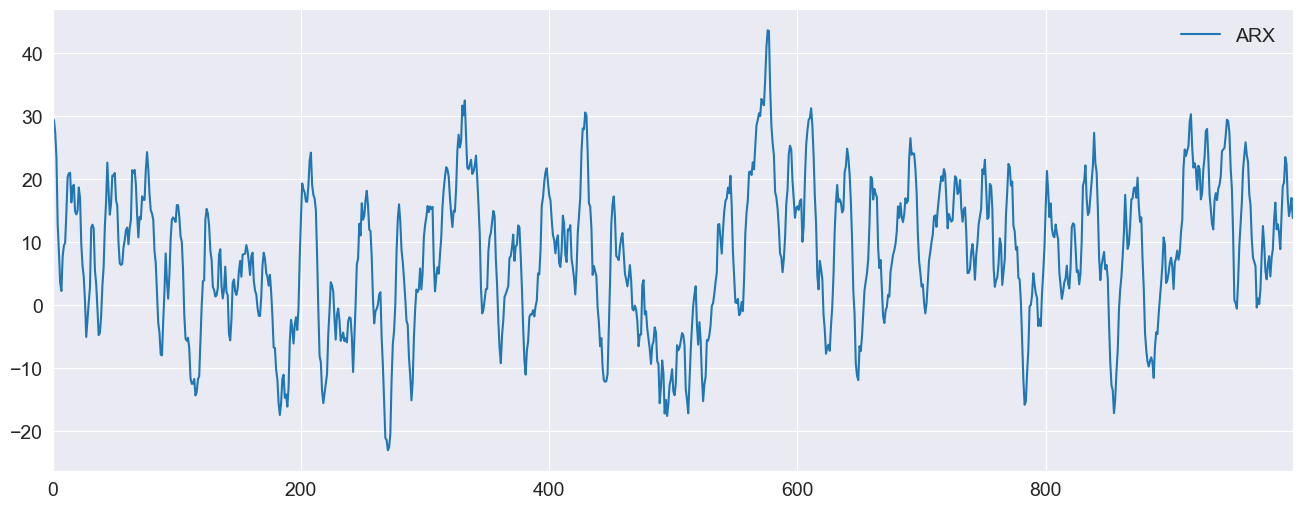
Forecasting the X values¶
The forecasts of \(Y\) depend on forecasts of \(X_0\) and \(X_1\). Both of these follow simple AR(1), and so we can construct the forecasts for all time horizons. Note that the value in position [i,j]
is the time-i
forecast for horizon j+1
.
[4]:
x0_oos = np.empty((1000, 10))
x1_oos = np.empty((1000, 10))
for i in range(10):
if i == 0:
last = x0
else:
last = x0_oos[:, i - 1]
x0_oos[:, i] = 1 + 0.8 * last
if i == 0:
last = x1
else:
last = x1_oos[:, i - 1]
x1_oos[:, i] = 2.5 + 0.5 * last
x1_oos[-1]
[4]:
array([6.34514725, 5.67257362, 5.33628681, 5.16814341, 5.0840717 ,
5.04203585, 5.02101793, 5.01050896, 5.00525448, 5.00262724])
Fitting the model¶
Next, the model is fit. The parameters are precisely estimated.
[5]:
exog = pd.DataFrame({"x0": x0, "x1": x1})
mod = arch_model(y, x=exog, mean="ARX", lags=1)
res = mod.fit(disp="off")
print(res.summary())
AR-X - GARCH Model Results
==============================================================================
Dep. Variable: data R-squared: 0.989
Mean Model: AR-X Adj. R-squared: 0.989
Vol Model: GARCH Log-Likelihood: -1374.68
Distribution: Normal AIC: 2763.35
Method: Maximum Likelihood BIC: 2797.70
No. Observations: 999
Date: Mon, Nov 04 2024 Df Residuals: 995
Time: 16:00:31 Df Model: 4
Mean Model
========================================================================
coef std err t P>|t| 95.0% Conf. Int.
------------------------------------------------------------------------
Const 3.0982 0.146 21.173 1.708e-99 [ 2.811, 3.385]
data[1] 0.7027 3.517e-03 199.807 0.000 [ 0.696, 0.710]
x0 1.9744 2.083e-02 94.805 0.000 [ 1.934, 2.015]
x1 -2.0069 2.504e-02 -80.160 0.000 [ -2.056, -1.958]
Volatility Model
==========================================================================
coef std err t P>|t| 95.0% Conf. Int.
--------------------------------------------------------------------------
omega 0.0913 3.193e-02 2.859 4.246e-03 [2.871e-02, 0.154]
alpha[1] 0.1510 3.318e-02 4.553 5.300e-06 [8.602e-02, 0.216]
beta[1] 0.7601 5.362e-02 14.175 1.313e-45 [ 0.655, 0.865]
==========================================================================
Covariance estimator: robust
Using a dict
¶
The first approach uses a dict to pass the two variables. The key consideration here is the the keys of the dictionary must exactly match the variable names (x0
and x1
here). The dictionary here contains only the final row of the forecast values since forecast
will only make forecasts beginning from the final in-sample observation by default.
Using DataFrame
¶
While these examples make use of NumPy arrays, these can be DataFrames
. This allows the index to be used to track the forecast origination point, which can be a helpful device.
[6]:
exog_fcast = {"x0": x0_oos[-1:], "x1": x1_oos[-1:]}
forecasts = res.forecast(horizon=10, x=exog_fcast)
forecasts.mean.T.plot()
[6]:
<Axes: >
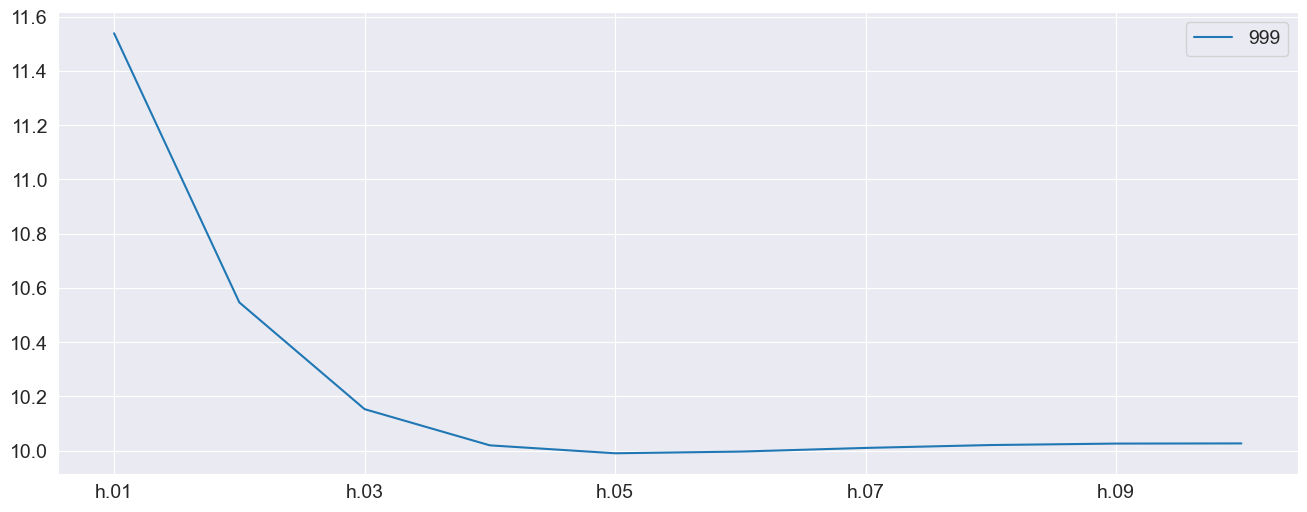
Using an array
¶
An array can alternatively be used. This frees the restriction on matching the variable names although the order must match instead. The forecast values are 2 (variables) by 1 (forecast) by 10 (horizon).
[7]:
exog_fcast = np.array([x0_oos[-1:], x1_oos[-1:]])
print(f"The shape is {exog_fcast.shape}")
array_forecasts = res.forecast(horizon=10, x=exog_fcast)
print(array_forecasts.mean - forecasts.mean)
The shape is (2, 1, 10)
h.01 h.02 h.03 h.04 h.05 h.06 h.07 h.08 h.09 h.10
999 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0
Producing multiple forecasts¶
forecast
can produce multiple forecasts using the same fit model. Here the model is fit to the first 500 observations and then forecasting for the remaining values are produced. It must be the case that the x
values passed for forecast
have the same number of rows as the table of forecasts produced.
[8]:
res = mod.fit(disp="off", last_obs=500)
exog_fcast = {"x0": x0_oos[-500:], "x1": x1_oos[-500:]}
multi_forecasts = res.forecast(start=500, horizon=10, x=exog_fcast)
multi_forecasts.mean.tail(10)
[8]:
h.01 | h.02 | h.03 | h.04 | h.05 | h.06 | h.07 | h.08 | h.09 | h.10 | |
---|---|---|---|---|---|---|---|---|---|---|
990 | 6.427409 | 5.678505 | 5.550445 | 5.756834 | 6.127391 | 6.562077 | 7.003670 | 7.421078 | 7.799049 | 8.131789 |
991 | 5.653403 | 5.212080 | 5.154577 | 5.359781 | 5.721542 | 6.159865 | 6.619864 | 7.066816 | 7.480757 | 7.851864 |
992 | 5.204390 | 4.751193 | 4.580281 | 4.689833 | 5.005348 | 5.444598 | 5.940164 | 6.444023 | 6.925402 | 7.366767 |
993 | 11.113016 | 11.420600 | 11.376927 | 11.198700 | 10.985574 | 10.780567 | 10.599841 | 10.447271 | 10.321479 | 10.219158 |
994 | 12.110336 | 12.031316 | 11.721126 | 11.366508 | 11.041428 | 10.768919 | 10.550430 | 10.379393 | 10.247223 | 10.145753 |
995 | 6.465793 | 6.492245 | 6.891241 | 7.382006 | 7.848326 | 8.249829 | 8.579405 | 8.843178 | 9.051418 | 9.214647 |
996 | 10.907973 | 12.063593 | 12.730623 | 13.004653 | 13.002989 | 12.827806 | 12.556598 | 12.243377 | 11.923287 | 11.617573 |
997 | 3.937348 | 4.132189 | 4.796268 | 5.575919 | 6.319854 | 6.972341 | 7.521071 | 7.972085 | 8.337976 | 8.632578 |
998 | -3.124113 | -1.765012 | 0.196835 | 2.124228 | 3.789854 | 5.145754 | 6.215404 | 7.044957 | 7.682544 | 8.170559 |
999 | -6.115248 | -4.480330 | -2.291303 | -0.131894 | 1.777204 | 3.379588 | 4.688134 | 5.740694 | 6.580315 | 7.247118 |
The plot of the final 5 forecast paths shows the the mean reversion of the process.
[9]:
_ = multi_forecasts.mean.tail().T.plot()
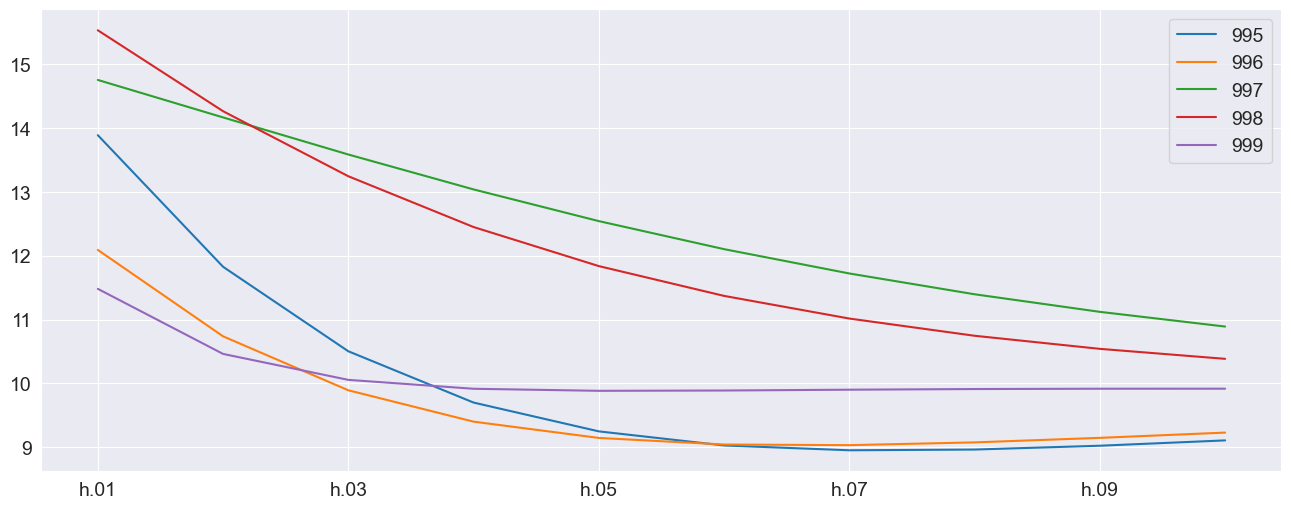
The previous example made use of dictionaries where each of the values was a 500 (number of forecasts) by 10 (horizon) array. The alternative format can be used where x
is a 3-d array with shape 2 (variables) by 500 (forecasts) by 10 (horizon).
[10]:
exog_fcast = np.array([x0_oos[-500:], x1_oos[-500:]])
print(exog_fcast.shape)
array_multi_forecasts = res.forecast(start=500, horizon=10, x=exog_fcast)
np.max(np.abs(array_multi_forecasts.mean - multi_forecasts.mean))
(2, 500, 10)
[10]:
np.float64(0.0)
x
input array sizes¶
While the natural shape of the x
data is the number of forecasts, it is also possible to pass an x
that has the same shape as the y
used to construct the model. The may simplify tracking the origin points of the forecast. Values are are not needed are ignored. In this example, the out-of-sample values are 2 by 1000 (original number of observations) by 10. Only the final 500 are used.
WARNING
Other sizes are not allowed. The size of the out-of-sample data must either match the original data size or the number of forecasts.
[11]:
exog_fcast = np.array([x0_oos, x1_oos])
print(exog_fcast.shape)
array_multi_forecasts = res.forecast(start=500, horizon=10, x=exog_fcast)
np.max(np.abs(array_multi_forecasts.mean - multi_forecasts.mean))
(2, 1000, 10)
[11]:
np.float64(0.0)
Special Cases with a single x
variable¶
When a model consists of a single exogenous regressor, then x
can be a 1-d or 2-d array (or Series
or DataFrame
).
[12]:
mod = arch_model(y, x=exog.iloc[:, :1], mean="ARX", lags=1)
res = mod.fit(disp="off")
print(res.summary())
AR-X - GARCH Model Results
==============================================================================
Dep. Variable: data R-squared: 0.933
Mean Model: AR-X Adj. R-squared: 0.933
Vol Model: GARCH Log-Likelihood: -2316.63
Distribution: Normal AIC: 4645.26
Method: Maximum Likelihood BIC: 4674.70
No. Observations: 999
Date: Mon, Nov 04 2024 Df Residuals: 996
Time: 16:00:32 Df Model: 3
Mean Model
========================================================================
coef std err t P>|t| 95.0% Conf. Int.
------------------------------------------------------------------------
Const -6.2299 0.257 -24.220 1.365e-129 [ -6.734, -5.726]
data[1] 0.7464 1.038e-02 71.885 0.000 [ 0.726, 0.767]
x0 1.7550 5.777e-02 30.379 1.050e-202 [ 1.642, 1.868]
Volatility Model
==========================================================================
coef std err t P>|t| 95.0% Conf. Int.
--------------------------------------------------------------------------
omega 5.4304 1.840 2.952 3.157e-03 [ 1.825, 9.036]
alpha[1] 0.1112 4.061e-02 2.739 6.156e-03 [3.165e-02, 0.191]
beta[1] 2.6000e-17 0.304 8.541e-17 1.000 [ -0.597, 0.597]
==========================================================================
Covariance estimator: robust
These two examples show that both formats can be used.
[13]:
forecast_1d = res.forecast(horizon=10, x=x0_oos[-1])
forecast_2d = res.forecast(horizon=10, x=x0_oos[-1:])
print(forecast_1d.mean - forecast_2d.mean)
## Simulation-forecasting
mod = arch_model(y, x=exog, mean="ARX", lags=1, power=1.0)
res = mod.fit(disp="off")
h.01 h.02 h.03 h.04 h.05 h.06 h.07 h.08 h.09 h.10
999 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0 0.0
Simulation¶
forecast
supports simulating paths. When forecasting a model with exogenous variables, the same value is used to in all mean paths. If you wish to also simulate the paths of the x
variables, these need to generated and then passed inside a loop.
Static out-of-sample x
¶
This first example shows that variance of the paths when the same x
values are used in the forecast. There is a sense the out-of-sample x
are treated as deterministic.
[14]:
x = {"x0": x0_oos[-1], "x1": x1_oos[-1]}
sim_fixedx = res.forecast(horizon=10, x=x, method="simulation", simulations=100)
sim_fixedx.simulations.values.std(1)
[14]:
array([[0.91626075, 1.1470658 , 1.40030468, 1.41469616, 1.26955774,
1.29757613, 1.29802358, 1.41217752, 1.52628177, 1.49453252]])
Simulating the out-of-sample x
¶
This example simulates distinct paths for the two exogenous variables and then simulates a single path. This is then repeated 100 times. We see that variance is much higher when we account for variation in the x data.
[15]:
from numpy.random import RandomState
def sim_ar1(params: np.ndarray, initial: float, horizon: int, rng: RandomState):
out = np.zeros(horizon)
shocks = rng.standard_normal(horizon)
out[0] = params[0] + params[1] * initial + shocks[0]
for i in range(1, horizon):
out[i] = params[0] + params[1] * out[i - 1] + shocks[i]
return out
simulations = []
rng = RandomState(20210301)
for i in range(100):
x0_sim = sim_ar1(np.array([1, 0.8]), x0.iloc[-1], 10, rng)
x1_sim = sim_ar1(np.array([2.5, 0.5]), x1.iloc[-1], 10, rng)
x = {"x0": x0_sim, "x1": x1_sim}
fcast = res.forecast(horizon=10, x=x, method="simulation", simulations=1)
simulations.append(fcast.simulations.values)
Finally the standard deviation is quite a bit larger. This is a most accurate value fo the long-run variance of the forecast residuals which should account for dynamics in the model and any exogenous regressors.
[16]:
joined = np.concatenate(simulations, 1)
joined.std(1)
[16]:
array([[3.08780599, 4.83111783, 6.40709867, 7.60549762, 8.40970494,
8.81096892, 8.59588943, 8.64276272, 8.97999513, 9.36824482]])
Conditional Mean Alignment vs. Forecast Alignment¶
When fitting a model with exogenous variables, the data are aligned so that the values in x[j]
are used to compute the conditional mean of y[j]
. For example, in the case of an AR(1)-X, the model is
We can recover the conditional mean by subtracting the residuals from the original data. When we do this we see that the conditional mean of observation 0 is missing since we need one lag of \(Y\) to fit the model.
[17]:
mod = arch_model(y, x=exog, mean="ARX", lags=1)
res = mod.fit(disp="off")
y - res.resid
[17]:
0 NaN
1 -15.555672
2 -15.225693
3 -13.113944
4 -4.405566
...
995 8.045397
996 9.178801
997 5.320586
998 -1.552152
999 -6.952927
Length: 1000, dtype: float64
Conditional Mean uses target alignment¶
When modeling the conditional mean in an AR-X, HAR-X, or LS model, the \(X\) data is target-aligned. This requires that when modeling the mean of y[t]
, the correct values of \(X\) must appear in x[t]
. Mathematically, the \(X\) matrix used when estimating a model should have the structure (using the Python indexing convention of a T-element data set having indices 0, 1, …, T-1):
forecast
uses origin alignment¶
Forecasting with \(X\) values aligns them differently. When producing a 1-step-ahead forecast for \(Y_{t+1}\) using information available at time \(t\), the \(X\) values used for this forecast must appear in for t
. This is needed since when once wants to produce true out-of-sample forecasts (see below), it must be the case that the final row of x
passed forecast
must all occur after the final time stamp of the most recent \(Y\) value. Mathematically, the \(X\)
matrix used in forecasting should have the following structure (using Python indexing convention so that a \(T\) observation dataset will have indices 0, 1, …, T-1).
where \(|\mathcal{F}_{s}\) is the time-\(s\) information set.
If you use the same x
value in the model when forecasting, you will see different values due to this alignment difference. Naively using the same x
values ie equivalent to setting
In general this would not be correct when forecasting, and will always produce forecasts that differ from the conditional mean. In order to recover the conditional mean using the forecast function, it is necessary to shift
the \(X\) values by -1, so that once shifted, the x
values will have the relationship
Here we shift the \(X\) data by -1
so x[s]
is treated as being in the information set for y[s-1]
. Also, note that the final forecast is NaN
. Conceptually this must be the case because the value of \(X\) at 999 should be ahead of 999 (i.e., at observation 1,000), and we do not have this value.
[18]:
exog_dict = {col: exog[[col]].shift(-1) for col in exog}
fcast = res.forecast(horizon=1, x=exog_dict, start=0)
fcast.mean
[18]:
h.1 | |
---|---|
0 | -15.555672 |
1 | -15.225693 |
2 | -13.113944 |
3 | -4.405566 |
4 | -1.300279 |
... | ... |
995 | 9.178801 |
996 | 5.320586 |
997 | -1.552152 |
998 | -6.952927 |
999 | NaN |
1000 rows × 1 columns
(Nearly) out-of-sample forecasts¶
These “in-sample” forecasts are not really forecasts at all but are just fitted values with a different alignment. If you want real (nearly) out-of-sample forecasts\(\dagger\), it is necessary to replace the actual values of \(X\) with their conditional expectation. This can be done by taking the fitted values from AR(1) models of the \(X\) variables.
\(\dagger\) These are not true out-of-sample since the parameters were estimated using data from that same range of indices where these forecasts target. True out-of-sample requires both using forecast \(X\) values and parameters estimated without the period being forecasted.
[19]:
res0 = ARX(exog["x0"], lags=1).fit()
res1 = ARX(exog["x1"], lags=1).fit()
forecast_x = pd.concat(
[res0.forecast(start=0).mean, res1.forecast(start=0).mean], axis=1
)
forecast_x.columns = ["x0f", "x1f"]
in_samp_forcast_exog = {"x0": forecast_x[["x0f"]], "x1": forecast_x[["x1f"]].shift(-1)}
fcast = res.forecast(horizon=1, x=in_samp_forcast_exog, start=0)
fcast.mean
[19]:
h.1 | |
---|---|
0 | -13.425601 |
1 | -13.914905 |
2 | -14.427516 |
3 | -8.946122 |
4 | 1.765684 |
... | ... |
995 | 6.687221 |
996 | 10.632919 |
997 | 2.182969 |
998 | -2.408569 |
999 | NaN |
1000 rows × 1 columns
True out-of-sample forecasts¶
In order to make a true out-of-sample prediction, we need the expected values of X
from the end of the data we have. These can be constructed by forecasting the two \(X\) variables and then passing these values as x
to forecast
.
[20]:
mod = arch_model(y, x=exog, mean="ARX", lags=1)
res = mod.fit(disp="off")
actual_x_oos = {
"x0": res0.forecast(horizon=10).mean,
"x1": res1.forecast(horizon=10).mean,
}
fcasts = res.forecast(horizon=10, x=actual_x_oos)
fcasts.mean
[20]:
h.01 | h.02 | h.03 | h.04 | h.05 | h.06 | h.07 | h.08 | h.09 | h.10 | |
---|---|---|---|---|---|---|---|---|---|---|
999 | -6.014692 | -4.324836 | -2.119601 | 0.036138 | 1.935561 | 3.529482 | 4.833372 | 5.885207 | 6.72714 | 7.398171 |